Introduction
Sphere Ramp Widget is a low code integration that allows you to seamlessly offer on-ramping and off-ramping services to your customers.
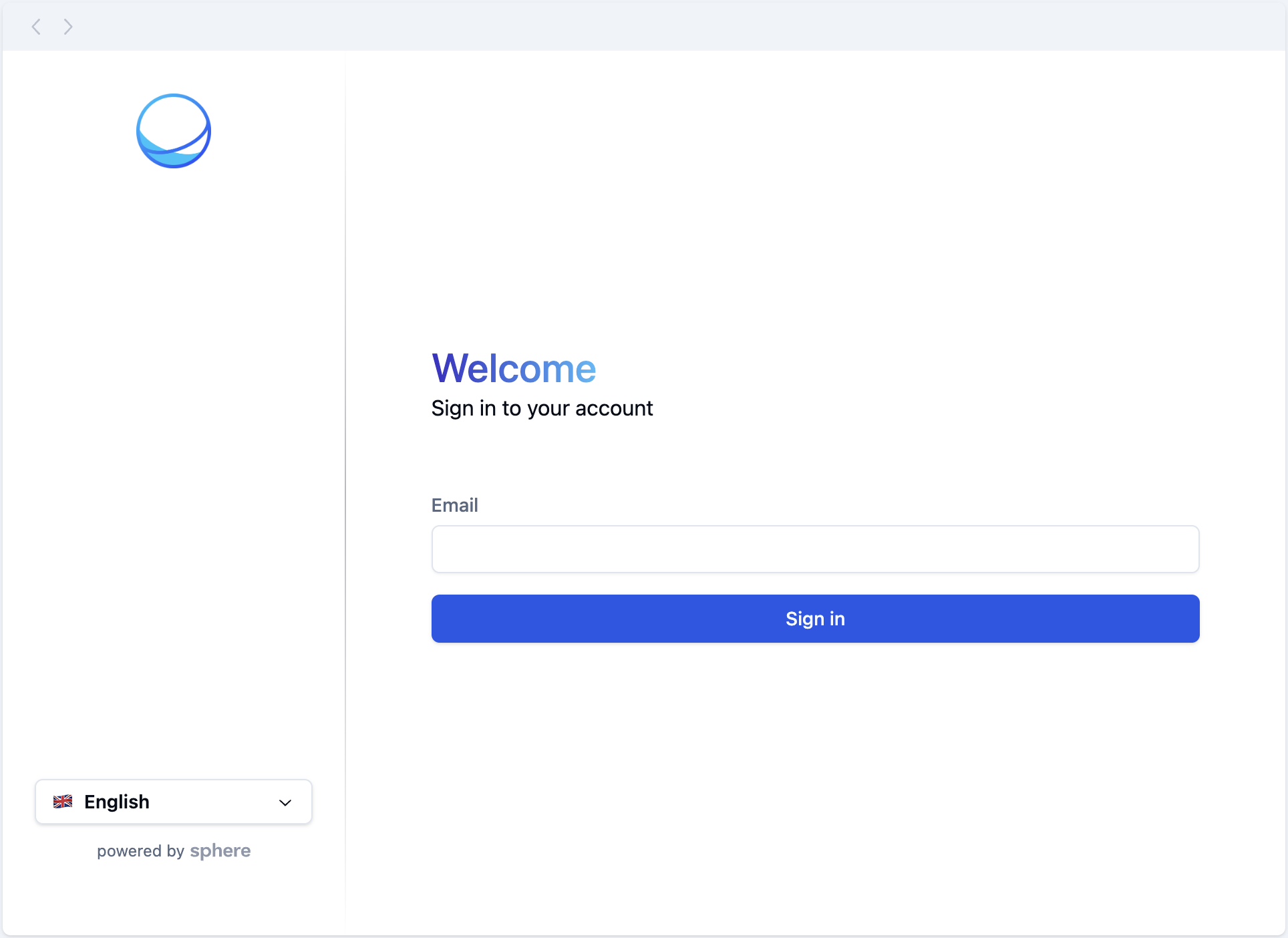
Overview
SphereRamp simplies the process of adding Sphere's comprehensive feature set to merchant applications. It provides everything needed right away, making the setup process effortless for engineers and eliminating the need for direct API connections.
Additionally, SphereRamp takes care of regulatory, security, and privacy concerns on behalf of the merchants.
Everything happens within the widget and Sphere's infrastructure, providing a secure and compliant service environment.
How it works
SphereRamp
is hosted on a CDN as a JavaScript bundle.
Installation comes down to initializing the widget within your app adding the asociated HTML element ID to the target element.
The following quickstart will show you everything you need to integrate.
Quickstart
A step-by-step guide for integrating SphereRamp into your application.
Getting your Application ID
Retrieve the application ID associated to your merchant in the dashboard.
You can find it on the Settings page:
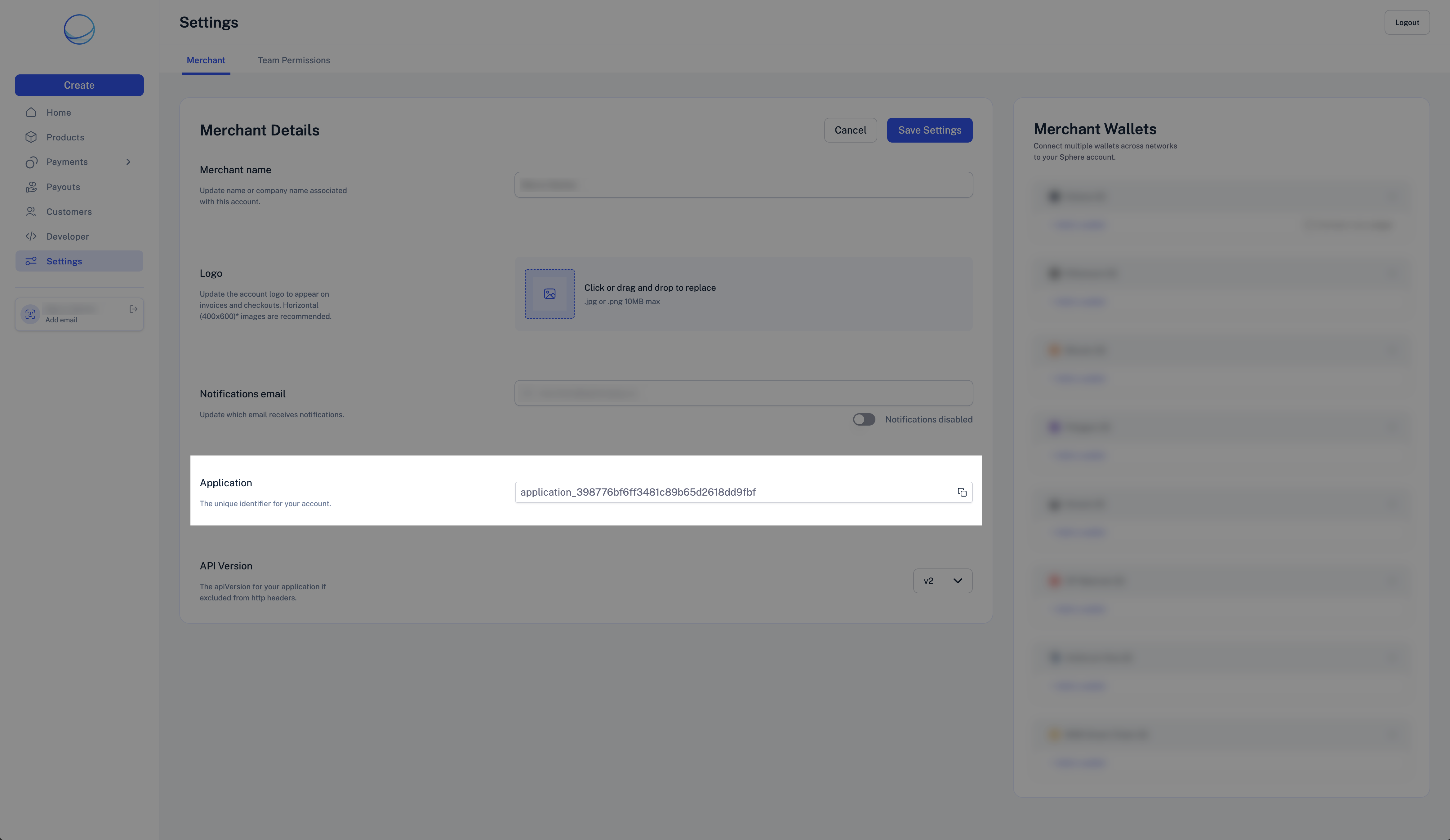
Live testing
Attention
Although designed for experimentation, the SphereRamp widget loaded under the
special /_ramp
route is still connected to our prod
environment.
You can experiment with SphereRamp without writing any code. Just visit the RampDemo page and provide your application ID as the applicationId
query parameter.
You can even enable the Debug Mode by adding debug=true as an extra parameter.
Setup
-
Import the SphereRamp script into your application.
- Add a
script
tag that points to the SphereRamp's bundled source code URL. - Ensure you have a root HTML element in place for SphereRamp to mount on.
HTML
<html> <head> <script type="module" crossorigin src="https://spherepay.co/packages/sphere-ramp/index.js"></script> </head> <body> <!-- ... Your App Code ... --> <div id="container"></div> <!-- ... Your App Code ... --> </body> </html>
- Add a
-
Assign an
onload
property to the script tag to call a function that initializes the widget.HTML<script type="module" crossorigin src="https://spherepay.co/packages/sphere-ramp/index.js" onload="initSphereRamp()" ></script>
-
Implement the initializer function.
JavaScriptfunction initSphereRamp() { new SphereRamp({ containerId: 'container', applicationId: 'YOUR_APPLICATION_ID', }) }
SphereRamp Properties
The SphereRamp
constructor accepts the following properties:
- Name
containerId
- Type
- string
- Required
- Required
- Description
The ID of the HTML element where the widget should be rendered.
- Name
applicationId
- Type
- string
- Required
- Required
- Description
Your application ID.
- Name
theme
- Type
- object | undefined
- Description
The theme object to customize the widget's appearance.
- Name
debug
- Type
- boolean | undefined
- Description
Whether to enable debug mode.
- Name
customerSupport
- Type
- boolean | undefined
- Description
Whether to enable Intercom.
- Name
allowKyb
- Type
- boolean | undefined
- Description
Whether to enable KYB.
Debug mode
Only for Development
Ensure this option is disabled before deploying to production.
SphereRamp provides a debug
mode for inspecting the application's behavior and exploring customization options.
To enable debug
mode, pass debug: true
to the configuration object of the SphereRamp instance:
new SphereRamp({
// ...
debug: true,
// ...
})
You then should be able to see the debugger beneath the widget:
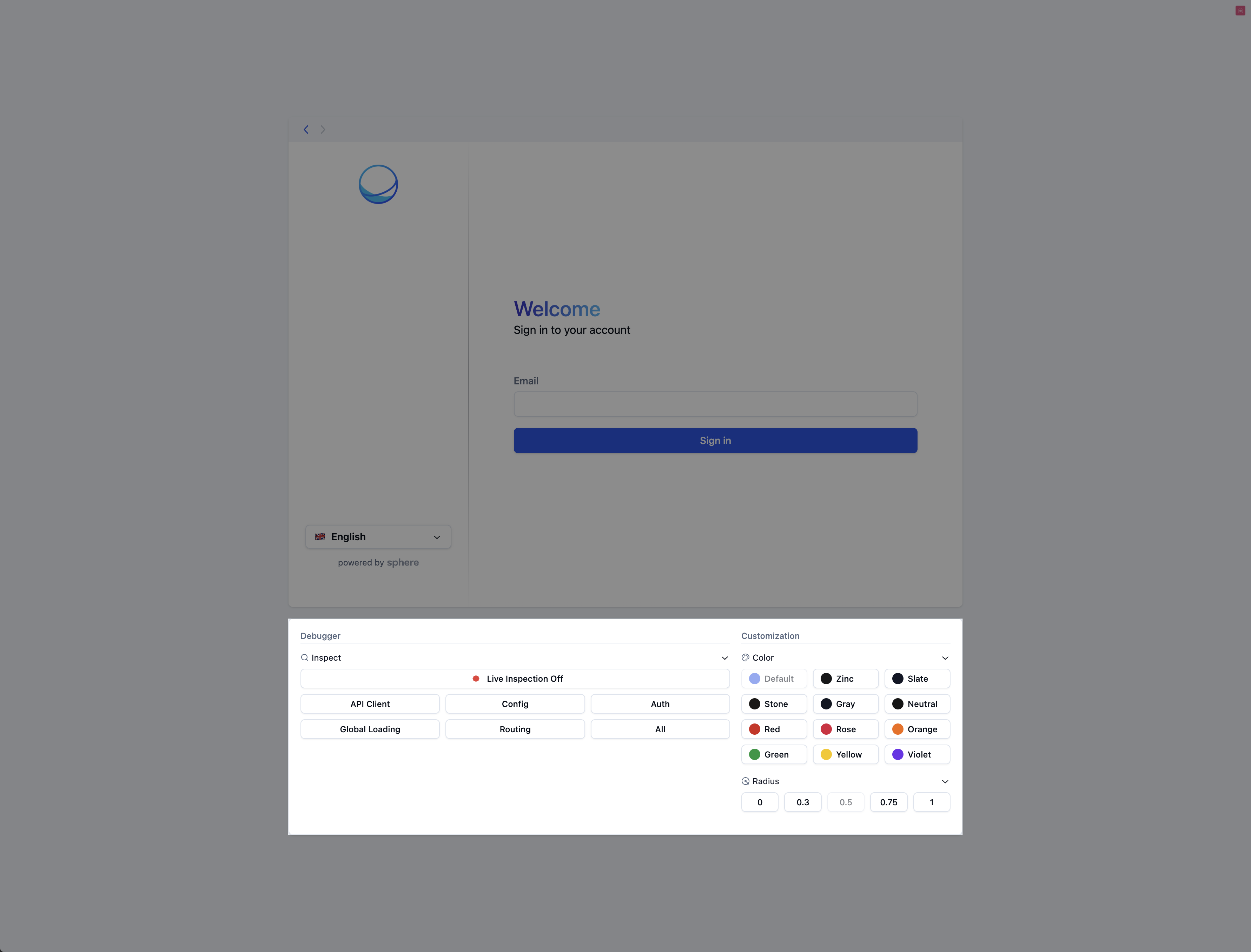
Further customization
SphereRamp allows merchants to comprehensively customize the look and feel of the widget, as well as branding assets such as the logo on the top left of it.
To start customizing, simply pass the theme
property to the configuration object. See Theming for all the available options.
Theming
Give SphereRamp the look and feel of your application.
Getting started
SphereRamp enables merchants to easily customize the widget to match their application's appearance. You do so by creating a theme
object and including it in the SphereRamp instance's configuration.
new SphereRamp({
theme: {
// ...
},
})
API Reference
/** The widget's primary color */
type ThemeColor =
| 'default'
| 'zinc'
| 'slate'
| 'stone'
| 'gray'
| 'neutral'
| 'red'
| 'rose'
| 'orange'
| 'green'
| 'yellow'
| 'violet'
/** The widget's border radius size */
type ThemeRadius = 'default' | 'none' | 'sm' | 'lg' | 'xl'
interface ThemeComponents {
/**
* The URL to a custom logo.
* We suggest using 56px x 56px images due to height constraints, but wider images should also work fine.
*/
logo: string
}
interface Theme {
color: ThemeColor
radius: ThemeRadius
components: ThemeComponents
}
Example
const theme = {
color: 'zinc',
radius: 'none',
components: {
logo: '...',
},
}
new SphereRamp({
theme,
})